Programming in Java
Object Oriented in Java
Advanced topics in Java
Tutorials
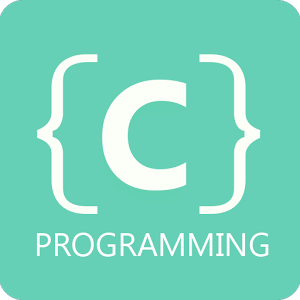
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Access Specifier in java
Java has four types of access specifiers and they are declared as following :
a. public :
public int a;
b. protected :
protected int b;
c. default (no specifier) :
int c;
d. private :
private int d;
All access modifiers can be understood with the following examples :
case 1 : Access variables within the class.
class A { public int a=10; protected int b=20; int c=30; private int d=40;
void show() { System.out.println("a="+a+" b="+b+" c="+c+" d="+d); } }
class CheckMain { public static void main(String args[]) { A obj= new A(); obj.show(); // all variables may be printed as they are used within the class. } }
case 2 : Access variable in the subclass of the same package.
class A
{
public static int a=10;
protected static int b=20;
static int c=30;
private static int d=40;
}
class B extends A
{
public static void main(String args[])
{
System.out.println(“a=”+a);
System.out.println(“b=”+b);
System.out.println(“c=”+c);
//System.out.println(“d=”+d); uncommenting this line will give error..
} //..as private variable cant be used here.
}
case 3 : Access variable in the non-subclass of the same package.
class A
{
public int a=10;
protected int b=20;
int c=30;
private int d=40;
}
class B
{
public static void main(String args[])
{
A obj= new A(); // need to create objects to access properties of another class without inheritance.
System.out.println(“a=”+obj.a);
System.out.println(“b=”+obj.b);
System.out.println(“c=”+obj.c);
//System.out.println(“d=”+obj.d); uncommenting this line will give error as private variable cant be used here.
}
}
case 4 : Access variable in the subclass of a different package.
package pkg1; class A { public static int a=10; protected static int b=20; static int c=30; private static int d=40; }
package pkg2; import pkg1.*; class B extends A { public static void main(String args[]) { System.out.println("a="+a); System.out.println("b="+b); //System.out.println("c="+c); uncommenting this line will give error as default variable can not be accessed outside package. //System.out.println("d="+d); uncommenting this line will give error as private variable is out of scope } }
case 5 : Access variable in the subclass of a different package.
package pkg1; class A { public int a=10; protected int b=20; int c=30; private int d=40; }
package pkg2; import pkg1.*; class B { public static void main(String args[]) { A obj= new A(); // need to create objects to access properties of another class without inheritance. System.out.println("a="+obj.a); // only public variable may be accessed here. //System.out.println("b="+obj.b); //System.out.println("c="+obj.c); //System.out.println("d="+obj.d); } }