Programming in Java
Object Oriented in Java
Advanced topics in Java
Modal Box
Tutorials
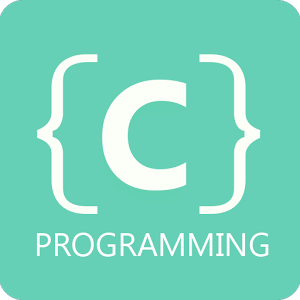
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
File Handling
The classes for file read and write are also present in java.io package .
Even java has many ways to read data from a given file, one of which we are using as following :
This program will display all the contents of a given file on the console .
import java.io.*;
public class ReadFileExample
{
public static void main(String args[])
{
try{
File file = new File("C:\\Users\\sharp\\Desktop\\test.txt");
FileReader fr=new FileReader(file);
BufferedReader br = new BufferedReader(fr);
String s=null;
while ((s = br.readLine()) != null)
System.out.println(s);
}
catch(Exception ex)
{
System.out.println(“Exception=”+ex);
}
}
}
Java program to write the contents into a file :
import java.io.*;
class FileWriterDemo
{
public static void main(String[] args) throws IOException
{
try{
File file = new File("C:\\Users\\sharp\\Desktop\\out.txt"); //file being created if it does not exist.
FileWriter fw=new FileWriter(file);
fw.write("Content to be written");
fw.close();
System.out.println("File written");
}
catch(Exception ex)
{
System.out.println("Exception is"+ex);
}
}
}
Video/ C Introduction
Watch video in full size