Basic Programs
If Else based Programs
Loop Based Programs
Array Based Programs
String Programs
Function Programs
Pointers Programs
Structure & File Handling Programs
Data Structure Programs
Array based programs
C Program to sort the array elements
#include <stdio.h> #include <conio.h> void main() { int a[10], i, j, temp; printf("enter 10 unsorted array elements"); for(i=0; i<10; i++) { scanf("%d", &a[i]); }
// logic to sort the elements in ascending order. for(i=0; i<9; i++) { for(j=i+1; j<10; j++) { if(a[j]<a[i]) // swap the elements if next elements are smaller than { // previous elements. temp=a[j]; a[j]=a[i]; a[i]=temp; } } }
printf("sorted array elements are \n"); for(i=0; i<10; i++) printf("%d ", a[i]); }
Output of the above program is as following.
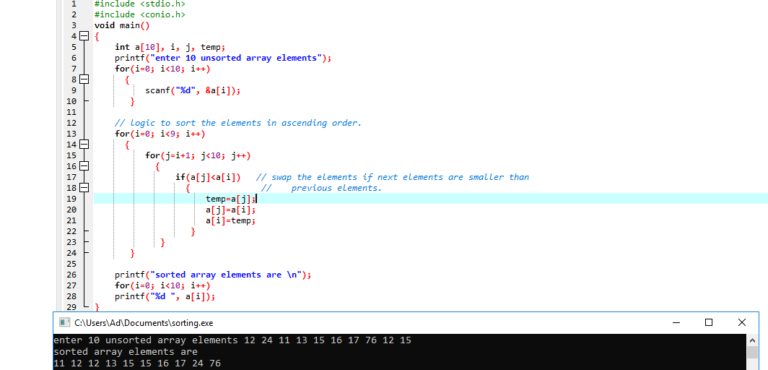
Selection Sort
#include <stdio.h> #include <conio.h> void main() { int a[10], i, j, temp,minindex; printf("enter 10 unsorted array elements"); for(i=0; i<10; i++) { scanf("%d", &a[i]); }
//selection sort logic to sort the elements in ascending order. for(i=0; i<9; i++) {
minindex=i; for(j=i+1; j<10; j++) { if(a[j]<a[minindex]) // swap the position if next element is smaller { minindex=j; }
} temp=a[i];
a[i]=a[minindex];
a[minindex]=temp; } printf("sorted elements are as follows\n"); for(i=0; i<10; i++)
{
printf("%d ", a[i]);
}
}
Output of the above program is as following.
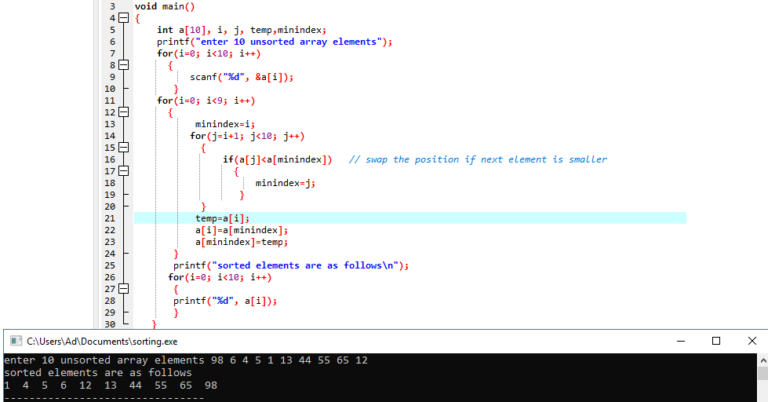
Bubble Sort
#include <stdio.h> #include <conio.h> void main() { int a[10],n=10, i, j, temp; printf("enter 10 unsorted array elements"); for(i=0; i<n; i++) { scanf("%d", &a[i]); }
// bubble sort logic to sort the elements in ascending order. for(i=0; i<n-1; i++) { for(j=0; j<n-1-i; j++) { if(a[j]>a[j+1]) { temp=a[j]; a[j]=a[j+1];
a[j+1]=temp; }
} } printf("sorted elements are as follows\n"); for(i=0; i<10; i++)
{
printf("%d ", a[i]);
}
}
Output of the above program is as following.
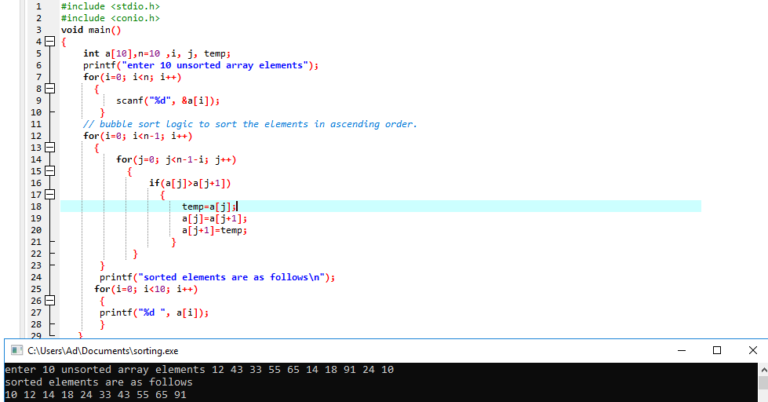
Insertion Sort
#include <stdio.h> #include <conio.h> void main() { int a[10],n=10, i, j, key; printf("enter 10 unsorted array elements"); for(i=0; i<n; i++) { scanf("%d", &a[i]); }
// insertion sort logic to sort the elements in ascending order. for(i=1; i<n; i++) { key=a[i]; j=i-1; while(j>=0 && a[j]>key) { if(a[j]>a[j+1]) { a[j+1]=a[j]; j=j-1;
} a[j+1]=key;
} } printf("sorted elements are as follows\n"); for(i=0; i<10; i++)
{
printf("%d ", a[i]);
}
}
Output of the above program is as following.
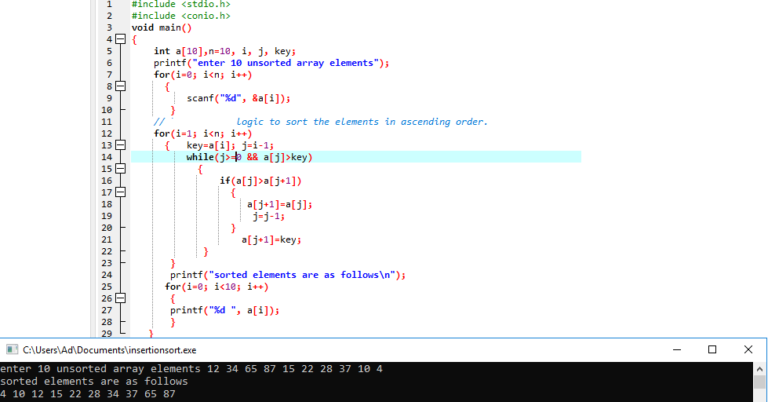
Video/ C Introduction
Watch video in full size