Programming in Java
Object Oriented in Java
Advanced topics in Java
Tutorials
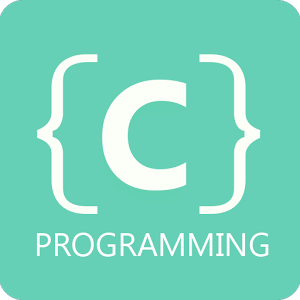
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Array in Java
Java array is an object which contains similar data type elements. In Java array a fixed no of elements can be stored.
Array in java is index-based, the index of first element in array is 0 while the last index is [size-1] .
Array may be single dimensional or multidimensional.
Single dimensional array :
It may be declared as following:
int a[]=new int[10];
Here name of the array is a and size of the array is 10.
So, index of the array elements will be 0-9.
How the array elements are accessed.
a[2]=55;
the above statement stores 5 value at 2 index of the array.
Declaration with initialization may be as following :
String name[]={“rahul“,”sunil“,”jyoti“};
Program to take 10 numbers from user as command line argument in an array and print the elements in reverse order.
class ArrayExample { public static void main(String args[]) { // declare an array of 10 size. int a[]= new int[10]; for(int i=0;i<10;i++) { //take input as command line arguments and convert it into integer. a[i]=Integer.parseInt(args[i]); } // print the array elements in reverse order. for(int i=9; i>=0; i--) { System.out.println("array elements: "+a[i]); } } }
Multi Dimensional (2D) Array:
int a[][]= new int[3][2];
where 3 is the no of row and 2 is no of column.
Eg: a[0][0] is the element at 0 row and 0 column.
In Java different number of columns may be created for each row ,
int a[][]= new int[3][]; // the [no. of columns] field is left empty here and is assigned below.
a[0]= new int[1];
a[1]= new int[2];
a[2]= new int[3];
In this case, every rows has different no. of columns or we can say zig zag array may be created.