Programming in Java
Object Oriented in Java
Advanced topics in Java
Tutorials
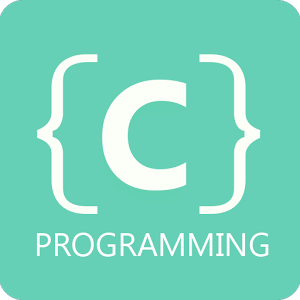
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
JDBC (Java Database Connectivity)
JDBC is a Java API to connect and execute the query with the database.
There are following four types of the drivers to connect in java with database :
1. JDBC-ODBC Bridge Driver
2. Native Driver
3. Network Protocol Driver
4. Thin Driver
How to connect with database using java
step 1 :
import java.sql.*;
step 2 :
Register the driver
//Register Ucan Access driver to connect java with MS-Access Database add Ucan Access Jar
Class.forName("net.ucanaccess.jdbc.UcanaccessDriver");
//Register MySQl Driver Add mysql-connector.jar here
Class.forName("com.mysql.jdbc.Driver");
step 3 :
Make the connection
Connection con= DriverManager.getConnection("jdbc:ucanaccess://E:\\datafile\\test.mdb;");//Establishing Connection with MS-Access Database
Connection con=DriverManager.getConnection("jdbc:mysql://localhost:3306/database","root","");//Establishing Connection with MySQl Database
step 4 :
Create Statement
Statement st=con.createStatement();
step 5 :
Execute the query
st.executeUpdate("insert or delete or update query");
ResultSet rs=st.executeQuery("select query here");
Complete Program to insert the student Data entered by user into a MySql table
import java.io.*;
import java.sql.*;
class StudentEntry{ public static void main(String[] args) {
try{
BufferedReader br= new BufferedReader(new InputStreamReader(System.in));
System.out.println(“enter student Name“); String name=br.readLine();
System.out.println(“enter the roll no”); int rollNo=Integer.parseInt(br.readLine());
Class.forName(“com.mysql.jdbc.Driver”);
Connection con=DriverManager.getConnection(“jdbc:mysql://localhost:3306/db”,”root”,””);
Statement st=con.createStatement();
st.executeUpdate(“insert into student values(“+rollNo+”,'”+name+”‘)”);// here student is the table name in database
System.out.println(“student registered”);
}
catch(Exception ex)
{
System.out.println(“exception”+ex);
}
}
}