Tkinter GUI Programming
Examples on Tkinter
Delete Records Example Tkinter
Using Python Tkinter GUI registration page may be created and records may be deleted from database after making the database connection.
Design Student Delete Page:
a) Design the Window Form
b) Add Control on Windows Form like label , TextField(Entry), Button etc
Delete Button Click
a)Call the function on button click and make database connectivity with mysql database .
b) delete the data from database on button click.
c) try the following code and see the output
from tkinter.ttk import *
from tkinter import *
import mysql.connector
from tkinter import messagebox
mydb=mysql.connector.connect(
host="localhost",
user="root",
password="",
database="sharp_db"
)
mycursor=mydb.cursor()
root=Tk()
root.title("Student Data")
root.geometry("1200x700")
photo=PhotoImage(file='image.png')
label12=Label(root,image=photo).grid(row=8,column=5)
label1=Label(root,text="RollNo",width=20,height=2,bg=" pink").grid(row=0,column=0)
label2=Label(root,text="First_Name",width=20,height=2,bg=" pink").grid(row=1,column=0)
label3=Label(root,text="Last_Name",width=20,height=2,bg=" pink").grid(row=2,column=0)
label4=Label(root,text="Phone_Number",width=20,height=2,bg=" pink").grid(row=3,column=0)
label5=Label(root,text="City",width=20,height=2,bg="pink"). grid(row=4,column=0)
label6=Label(root,text="State",width=20,height=2,bg="pink"). grid(row=5,column=0)
label7=Label(root,text="Age",width=20,height=2,bg="pink"). grid(row=6,column=0)
label8=Label(root,width=10,height=2).grid(row=7,column=2)
label9=Label(root,width=10,height=2).grid(row=7,column=4)
label10=Label(root,width=10,height=2).grid(row=7,column=6)
label11=Label(root,width=10,height=2).grid(row=7,column=8)
e1=Entry(root,width=30,borderwidth=8)
e1.grid(row=0,column=1)
e2=Entry(root,width=30,borderwidth=8)
e2.grid(row=1,column=1)
e3=Entry(root,width=30,borderwidth=8)
e3.grid(row=2,column=1)
e4=Entry(root,width=30,borderwidth=8)
e4.grid(row=3,column=1)
e5=Entry(root,width=30,borderwidth=8)
e5.grid(row=4,column=1)
e6=Entry(root,width=30,borderwidth=8)
e6.grid(row=5,column=1)
e7=Entry(root,width=30,borderwidth=8)
e7.grid(row=6,column=1)
def Delete():
RollNo=e1.get()
Delete="delete from student where RollNo='%s'" %(RollNo)
mycursor.execute(Delete)
mydb.commit()
messagebox.showinfo("Information","Record Deleted")
e1.delete(0,END)
e2.delete(0, END)
e3.delete(0, END)
e4.delete(0, END)
e5.delete(0, END)
e6.delete(0, END)
e7.delete(0,END)
button2=Button(root,text="Delete",width=10,height=2,comm and=Delete).grid(row=7,column= 1)
root.mainloop()
when we run the above code output is:
Click Here
Tutorials
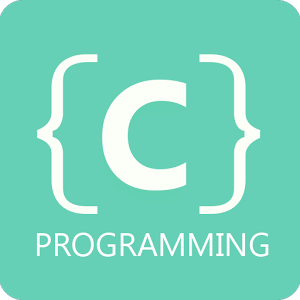
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Video/ C Introduction
Watch video in full size