Introduction to Python
Collection in Python
Object Oriented python
Python With MYSQL And Excel
Python GUI
Programs in Python
- Swap two number
- Calculate the area
- Even Odd or Zero
- Largest ,Middle and Smallest Number
- Calculate Telephone Bill
- Print Table of The given Number
- Factorial of the number
- Reverse and check number is palindrome
- check number is prime , armstrong
- Program to Print the given patterns
- Guess A Number Game using Random
Inheritance in Python
Inheritance is the powerful property of object oriented programming through which the features of a class are derived into its sub class.
or we can Say “inheritance is the super class -sub class or parent class-child class relationship.
How inheritance is achieved in python.
Single Inheritance:
class A: def display(self): print("this is base class function") class B(A): # how class is inherited def show(self): print("this is sub class function") a1=A() a1.display() # A clas object may call only A class functions. b1=B() b1.display() b1.show() # B class object may call B class as well as A class object.
Output:
this is base class function
this is base class function
this is sub class function
Multilevel inheritance in python:
class A: def test1(self): print("A class function") class B(A): def test2(self): print("B class function") class C(B): # multilevel inheritance def test3(self): print("C class function") c1=C() c1.test1() #c class object may also call A and B class functions. c1.test2() c1.test3()
Output:
A class function
B class function
C class function
Multiple inheritance in python:
class A: def test1(self): print("A class function") class B(A): def test2(self): print("B class function")
class C(A,B): #C class inherites both A & B classes def test3(self): print("C class function") c1=C() c1.test1() #c class object may also call A and B class functions. c1.test2() c1.test3()
Output:
A class function
B class function
C class function
Click Here
Tutorials
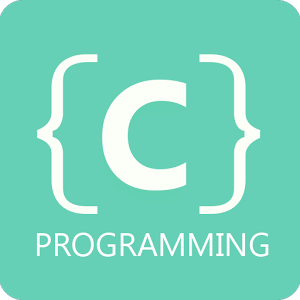
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Video/ C Introduction
Watch video in full size